Code Garden
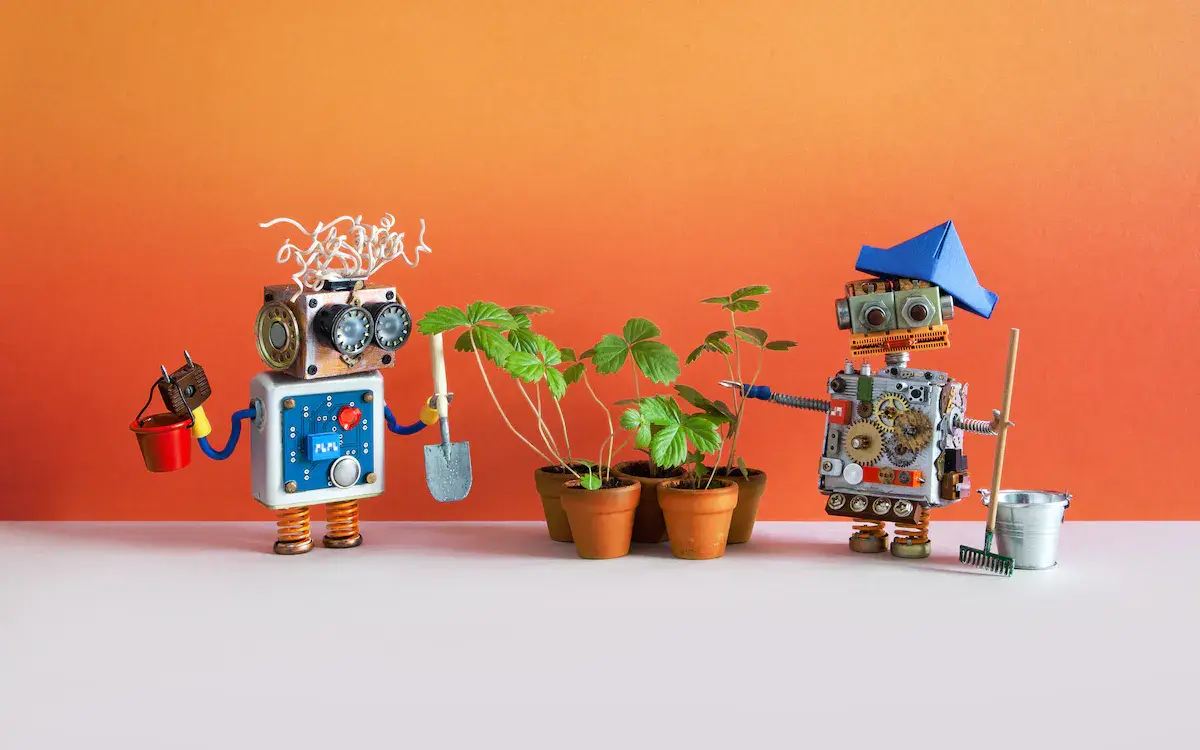
The start of the next course for my masters class began this week. In the preparation work we were asked to set up a "portfolio" of files that we would have to refer back to. Examples of good code as well as interesting errors stored away for those times when you need to take a look at how you might have solved a problem well, or some particular thing that stumped you. There has been a lot of talk around blogs and using your blog as a "digital garden". It's a way to organize your writings without worrying as much about the presentation at the end. I found the idea of doing the same thing with code to be valuable. The thought of having a library of code snippets of ideas and fundamentals that I learn to refer back to seems to be a great gift to my future self, you know the one that I constantly push responsibilities off. I think I owe him at least that.
So what exactly then do you plant in yout code garden? For class it will be specified by the assignments that we do. Things like good examples of loops, sentinel controlled structures, nested loops, and similar topics from the class course work. What about outsde of class? I hope to add things that I constantly flex my google skills to remind myself how to do. A component library of code samples so to speak. Open sourcing the learnings of both my course work as well as my daily work and side project work.
Besides the code garden we finally delved into the subject of pointers in class. Ironically this came at the same time as I began to really work with graphql code written in Go which takes advantage of pointers. In this post we will be using C for the example code, as that's what we are using in the masters class. So what is a pointer? A pointer is a reference to the address in memory of a value. Let's take a look at how we would create one.
// we declare and initialize a variable with the value of 10
int integer = 10;
// we declare a pointer variable that will reference an int
int *int_pointer;
The asterisk is the key part here. It signifies something special about the variable. It makes the type of int_pointer a pointer to int. So then what do we do with this new pointer?
// we can assign the address of integer to int_pointer
int_pointer = &integer;
Here we are utilizing the &
or address operator to get the address of the integer value and assign that to the int_pointer
. Now let's take a look at how you would use that pointer.
// we need a variable that can hold an int value
int y;
// we can then use our reference to initialize the value of y
y = *int_pointer;
printf("%d", y); // will print out 10
The *
used in this case is refered to as the indirection operator. It basically uses the reference to indirectly assign the value assigned to integer
earlier in the code to the new variable y
. All of this seems interesting but not very useful yet. So where does the values of using pointers really start to pay off? In the next post in this series we will take a look at using pointers with structs and see how they can start to become much more useful than just using the actual values.